This example is modified from ble_app_template
located in:
nRF5_SDK_14.2.0_17b948a\nRF5_SDK_14.2.0_17b948a\examples\ble_peripheral\ble_app_template
Board:nRF52840/SDK14/S140
STEP 0. project config
add these files into nrf_library
"app_uart_fifo.c"
"app_fifo.c"
"retarget.c"
located in :
nRF5_SDK_14.2.0_17b948a\components\libraries\uart
nRF5_SDK_14.2.0_17b948a\components\libraries\fifo
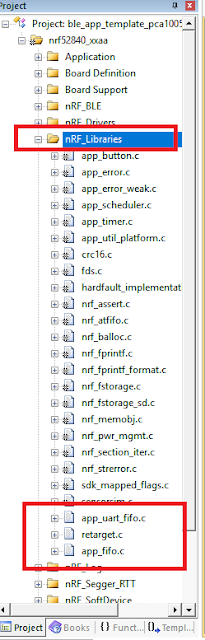
next include path to project option
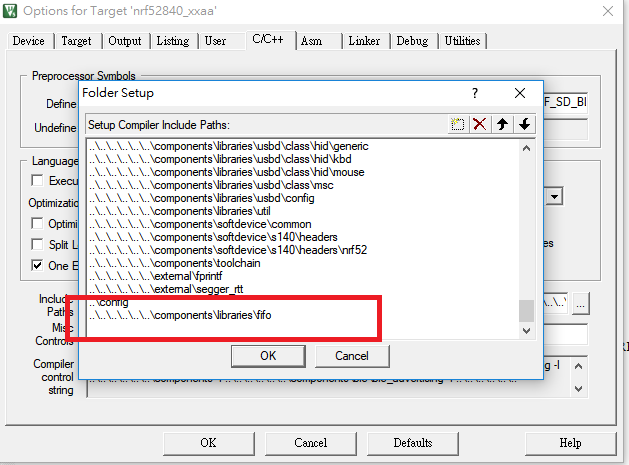
STEP 1. modify "sdk_config"
I suggest first open sdk_config.h Text Editor
add these about line 3004 (after nRF_Libraries )
//========================================================== // <q> APP_FIFO_ENABLED - app_fifo - Software FIFO implementation #ifndef APP_FIFO_ENABLED #define APP_FIFO_ENABLED 1 #endif
then add these about line 3080 (after APP_TWI_ENABLED )
// <e> APP_UART_ENABLED - app_uart - UART driver //========================================================== #ifndef APP_UART_ENABLED #define APP_UART_ENABLED 1 #endif // <o> APP_UART_DRIVER_INSTANCE - UART instance used // <0=> 0 #ifndef APP_UART_DRIVER_INSTANCE #define APP_UART_DRIVER_INSTANCE 0 #endif
then add these about line 3506(after NRF_QUEUE_ENABLED)
// <q> RETARGET_ENABLED - retarget - Retargeting stdio functions #ifndef RETARGET_ENABLED #define RETARGET_ENABLED 1 #endif
Then open sdk_config.h Configuration Wizard
you can see:
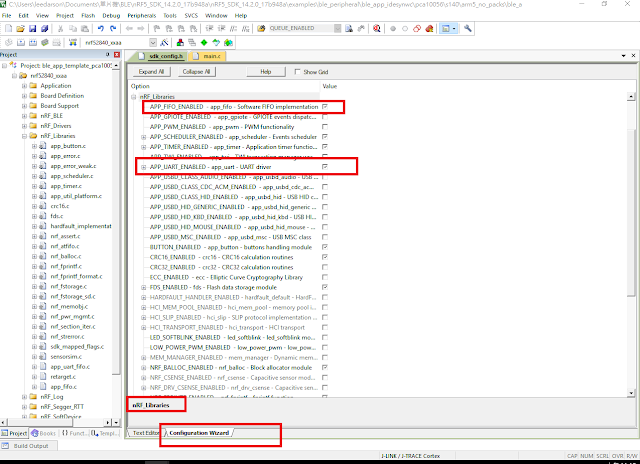
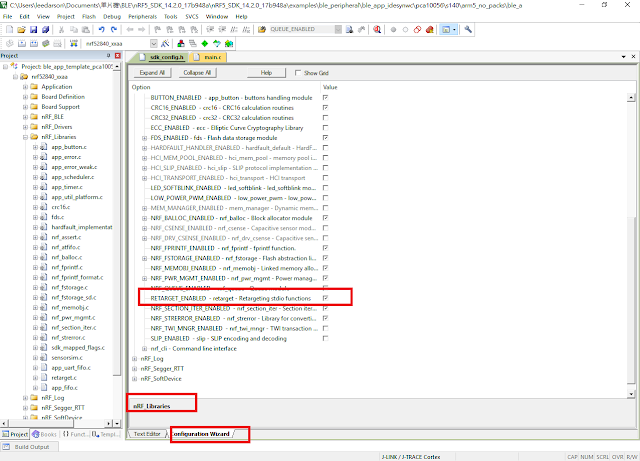
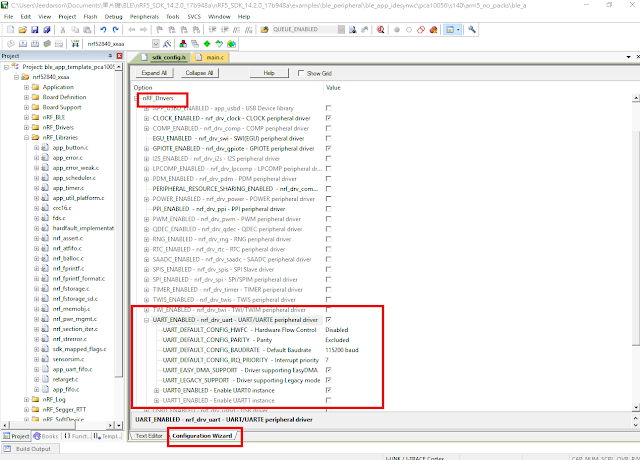
but if you prefer to add code , please add in sdk_config.h Text Editor
about line 2724 (after TWI_ENABLE)
// <e> UART_ENABLED - nrf_drv_uart - UART/UARTE peripheral driver //========================================================== #ifndef UART_ENABLED #define UART_ENABLED 1 #endif // <o> UART_DEFAULT_CONFIG_HWFC - Hardware Flow Control // <0=> Disabled // <1=> Enabled #ifndef UART_DEFAULT_CONFIG_HWFC #define UART_DEFAULT_CONFIG_HWFC 0 #endif // <o> UART_DEFAULT_CONFIG_PARITY - Parity // <0=> Excluded // <14=> Included #ifndef UART_DEFAULT_CONFIG_PARITY #define UART_DEFAULT_CONFIG_PARITY 0 #endif // <o> UART_DEFAULT_CONFIG_BAUDRATE - Default Baudrate // <323584=> 1200 baud // <643072=> 2400 baud // <1290240=> 4800 baud // <2576384=> 9600 baud // <3862528=> 14400 baud // <5152768=> 19200 baud // <7716864=> 28800 baud // <10289152=> 38400 baud // <15400960=> 57600 baud // <20615168=> 76800 baud // <30801920=> 115200 baud // <61865984=> 230400 baud // <67108864=> 250000 baud // <121634816=> 460800 baud // <251658240=> 921600 baud // <268435456=> 1000000 baud #ifndef UART_DEFAULT_CONFIG_BAUDRATE #define UART_DEFAULT_CONFIG_BAUDRATE 30801920 #endif // <o> UART_DEFAULT_CONFIG_IRQ_PRIORITY - Interrupt priority // <i> Priorities 0,2 (nRF51) and 0,1,4,5 (nRF52) are reserved for SoftDevice // <0=> 0 (highest) // <1=> 1 // <2=> 2 // <3=> 3 // <4=> 4 // <5=> 5 // <6=> 6 // <7=> 7 #ifndef UART_DEFAULT_CONFIG_IRQ_PRIORITY #define UART_DEFAULT_CONFIG_IRQ_PRIORITY 7 #endif // <q> UART_EASY_DMA_SUPPORT - Driver supporting EasyDMA #ifndef UART_EASY_DMA_SUPPORT #define UART_EASY_DMA_SUPPORT 1 #endif // <q> UART_LEGACY_SUPPORT - Driver supporting Legacy mode #ifndef UART_LEGACY_SUPPORT #define UART_LEGACY_SUPPORT 1 #endif // <e> UART0_ENABLED - Enable UART0 instance //========================================================== #ifndef UART0_ENABLED #define UART0_ENABLED 1 #endif // <q> UART0_CONFIG_USE_EASY_DMA - Default setting for using EasyDMA #ifndef UART0_CONFIG_USE_EASY_DMA #define UART0_CONFIG_USE_EASY_DMA 1 #endif // </e> // <e> UART1_ENABLED - Enable UART1 instance //========================================================== #ifndef UART1_ENABLED #define UART1_ENABLED 0 #endif // <q> UART1_CONFIG_USE_EASY_DMA - Default setting for using EasyDMA #ifndef UART1_CONFIG_USE_EASY_DMA #define UART1_CONFIG_USE_EASY_DMA 1 #endif // </e> // </e> // <e> USBD_ENABLED - nrf_drv_usbd - USB driver //========================================================== #ifndef USBD_ENABLED #define USBD_ENABLED 0 #endif // <o> USBD_CONFIG_IRQ_PRIORITY - Interrupt priority // <i> Priorities 0,2 (nRF51) and 0,1,4,5 (nRF52) are reserved for SoftDevice // <0=> 0 (highest) // <1=> 1 // <2=> 2 // <3=> 3 // <4=> 4 // <5=> 5 // <6=> 6 // <7=> 7 #ifndef USBD_CONFIG_IRQ_PRIORITY #define USBD_CONFIG_IRQ_PRIORITY 7 #endif
STEP 2. disable NRF_LOG in UART
open sdk_config.h Configuration Wizard and check nrf_log Logger
disable them all
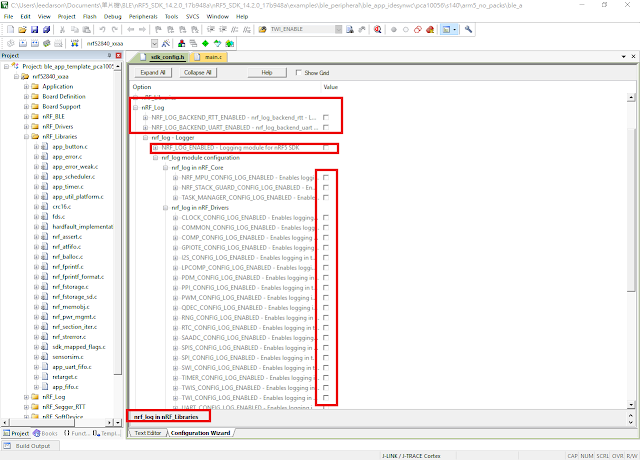
STEP 3. modify main.c
add these include & #define in main.c
#include "app_uart.h" #if defined (UART_PRESENT) #include "nrf_uart.h" #endif #if defined (UARTE_PRESENT) #include "nrf_uarte.h" #endif #define UART_HWFC APP_UART_FLOW_CONTROL_DISABLED #define UART_TX_BUF_SIZE 256 /**< UART TX buffer size. */ #define UART_RX_BUF_SIZE 256 /**< UART RX buffer size. */
add below code after buttons_leds_init( ) function :
void uart_error_handle(app_uart_evt_t * p_event) { if (p_event->evt_type == APP_UART_COMMUNICATION_ERROR) { APP_ERROR_HANDLER(p_event->data.error_communication); } else if (p_event->evt_type == APP_UART_FIFO_ERROR) { APP_ERROR_HANDLER(p_event->data.error_code); } } static void uart_init(void) { uint32_t err_code; const app_uart_comm_params_t comm_params = { RX_PIN_NUMBER, TX_PIN_NUMBER, RTS_PIN_NUMBER, CTS_PIN_NUMBER, UART_HWFC, false, NRF_UART_BAUDRATE_115200 }; APP_UART_FIFO_INIT(&comm_params, UART_RX_BUF_SIZE, UART_TX_BUF_SIZE, uart_error_handle, APP_IRQ_PRIORITY_LOWEST, err_code); APP_ERROR_CHECK(err_code); }
in main( ) function
please #if(0) log_init( ) and add uart_init( ) as this :
int main(void) { bool erase_bonds; // Initialize. #if(0) log_init(); #endif timers_init(); buttons_leds_init(&erase_bonds); uart_init(); printf("agathakuan\r\n"); printf("system start!!\r\n"); ble_stack_init(); gap_params_init(); gatt_init(); advertising_init(); services_init(); conn_params_init(); peer_manager_init(); // Start execution. NRF_LOG_INFO("Template example started."); application_timers_start(); advertising_start(erase_bonds); // Enter main loop. for (;;) { if (NRF_LOG_PROCESS() == false) { power_manage(); } } }
program and reset your device, then open your COM port, you can see:
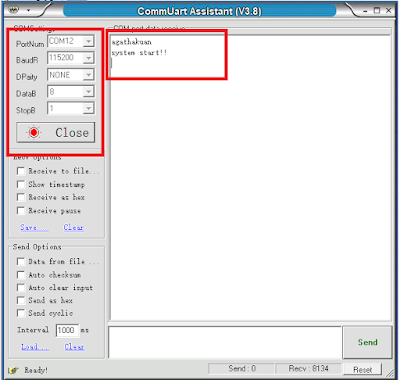
NOTE:
1. in SDK14, the developer must assign UART, PWM,WDT...etc in sdk_config.h
otherwise, even they include all the paths and files needed, they still can not open UART.
2. if you activate NRF_LOG_BACKEND_UART, you should not use printf( ), else it will cause system fatal error.
3.The way to activate UART in SDK14 is NOT the same as SDK12
REFERENCE:
http://gaiger-programming.blogspot.tw/2016/12/nrf51-make-printf-works-well.html?m=1
http://programnoteagatha.blogspot.tw/2017/05/nrf52-printf-in-sdk12.html